07 Addition rule for probability
Contents
07 Addition rule for probability#
%%html
<iframe width="700" height="400" src="https://www.youtube.com/embed/QE2uR6Z-NcU/" frameborder="0" allowfullscreen></iframe>
import numpy as np
import pandas as pd
from pandas import Series, DataFrame
import matplotlib.pyplot as plt
from matplotlib_venn import venn2
import seaborn as sns
bag = 8 * ['Green-Cube'] + 9 * ['Green-Sphere'] + 5 * ['Yellow-Cube'] + 7 * ['Yellow-Sphere']
def P(cond, total_lst):
cond_lst = list(filter(cond, total_lst))
return len(cond_lst) / len(total_lst), cond_lst
# P(Cube)
p_cube, p_cube_lst = P(lambda x: x.endswith('Cube'), bag)
print(p_cube)
0.4482758620689655
# P(Yellow)
p_yellow, p_yellow_lst = P(lambda x: x.startswith('Yellow'), bag)
print(p_yellow)
0.41379310344827586
# P(Yellow And Cube)
p_yellow_and_cube, _ = P(lambda x: x.startswith('Yellow') and x.endswith('Cube'), bag)
print(p_yellow_and_cube)
0.1724137931034483
# P(Yellow or Cube)
p_yellow_or_cube, _ = P(lambda x: x.startswith('Yellow') or x.endswith('Cube'), bag)
print(p_yellow_or_cube)
0.6896551724137931
P(A OR B) = P(A) + P(B) - P(A AND B)#
# P(Yellow or Cube) = P(Yellow) + P(Cube) - P(Yellow And Cube)
p_yellow_or_cube == p_yellow + (p_cube - p_yellow_and_cube) # find the reason why
True
def intersection(lst1, lst2):
lst3 = [value for value in lst1 if value in lst2]
return lst3
p_yellow_interset_p_cube_lst = [val for val in p_yellow_lst if val in p_cube_lst]
venn2(subsets=[len(p_cube_lst),
len(p_yellow_lst),
len(p_yellow_interset_p_cube_lst)],
set_labels=['Cubes', 'Yellow'],
set_colors=['Blue', 'Yellow'])
<matplotlib_venn._common.VennDiagram at 0x7feabbbc6e50>
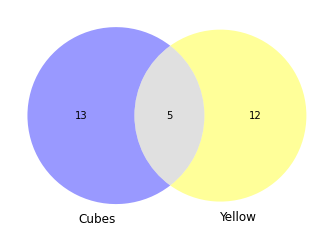