05 Techniques for generating a simple random sample
05 Techniques for generating a simple random sample#
%%html
<iframe width="700" height="400" src="https://www.youtube.com/embed/acfjqWTwee0/" frameborder="0" allowfullscreen></iframe>
import random
import numpy as np
import pandas as pd
from pandas import Series, DataFrame
import matplotlib.pyplot as plt
import seaborn as sns
from scipy import stats
# Generate A Random Number From The Normal Distribution
np.random.normal()
-1.0227078604110735
# Generate Four Random Numbers From The Normal Distribution
np_normdist = np.random.normal(size=4)
print(np_normdist)
sns.distplot(np_normdist, hist=False)
[-0.1058692 0.72574354 -0.41925714 -0.51047041]
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `kdeplot` (an axes-level function for kernel density plots).
warnings.warn(msg, FutureWarning)
<AxesSubplot:ylabel='Density'>
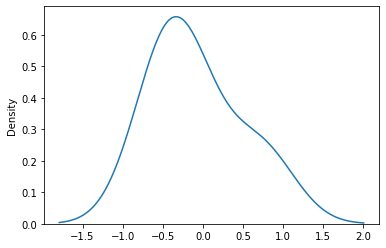
st_normdist = stats.norm.rvs(size=4)
print(np_normdist)
sns.distplot(st_normdist, hist=False)
[-0.1058692 0.72574354 -0.41925714 -0.51047041]
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `kdeplot` (an axes-level function for kernel density plots).
warnings.warn(msg, FutureWarning)
<AxesSubplot:ylabel='Density'>
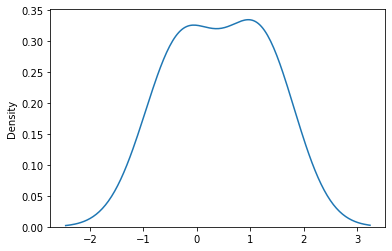
# Generate A Random Number From The Uniform Distribution
np.random.uniform()
0.9256937634924077
# Generate Four Random Numbers From The Uniform Distribution
unidist = np.random.uniform(size=4)
sns.distplot(unidist)
/opt/hostedtoolcache/Python/3.9.13/x64/lib/python3.9/site-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
<AxesSubplot:ylabel='Density'>
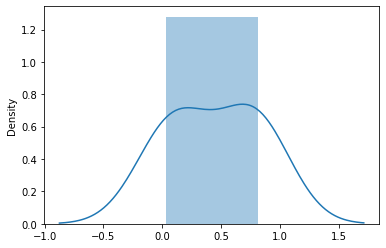
# Generate Four Random Integers Between 1 and 100
np.random.randint(low=1, high=100, size=4)
array([50, 38, 84, 74])
# Choice
A =['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
print(random.choice(A))
print(np.random.choice(A))
K
G
random.sample(A, 5)
['G', 'T', 'J', 'L', 'D']